
What is an API: The 101 Guide for Developers and Architects
Back to basics: This guide explores the fundamentals of APIs, how they work, their different types, and the benefits they bring to the software development process.
An API, or Application Programming Interface, is a critical component in modern software development and architecture.
APIs allow different systems to communicate and exchange data, making them the foundation of many applications today.
As businesses move towards microservices and cloud-based solutions, understanding APIs is more important than ever for developers and architects.
This guide explores the fundamentals of APIs, how they work, their different types, and the benefits they bring to the software development process.
What is an API?
An API, short for Application Programming Interface, is a set of defined rules that enable software applications to interact with each other.
APIs expose some part of an application’s functionality to other software systems, allowing them to request and retrieve data.
Essentially, APIs serve as a bridge between different software programs, enabling them to communicate without needing to understand each other’s internal workings.
APIs can be compared to a waiter in a restaurant.
Just as the waiter takes your order and delivers it to the kitchen, an API takes a request from one system (the client) and delivers it to another (the server).
The server processes the request and sends a response back to the client, just as the waiter delivers your food to the table.
This abstraction allows developers to build complex systems that leverage external data or services without knowing the intricate details of how those systems work internally.
How Do APIs Work?
APIs function as intermediaries that allow two or more software systems to communicate with each other.
This communication typically follows a request-response pattern.
A client (such as a web application or mobile app) makes a request to an API, specifying the needed data or action.
The API processes this request and responds with the requested data or an acknowledgment of the action.
For example, when you use a weather app on your phone, the app sends a request to a weather API. The API retrieves the latest weather data from a remote server and sends it back to the app, which then displays the information to you. This entire interaction happens in the background, and the user only sees the result—current weather updates.
APIs primarily use HTTP requests like GET, POST, PUT, and DELETE to communicate between the client and the server.
Each request includes parameters (or data) that tell the API exactly what information is being requested or what action is to be performed. The server processes the request and returns the appropriate response, often in a format like JSON or XML.
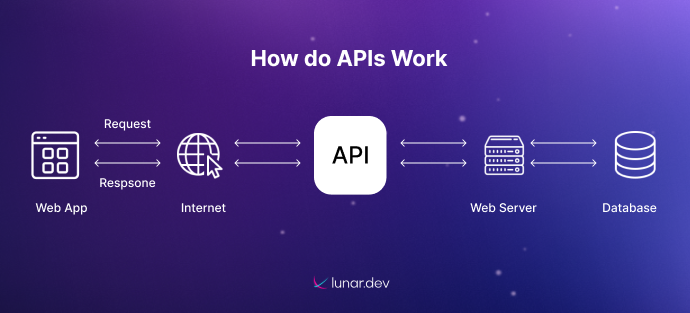
Types of APIs
There are several types of APIs that serve different purposes in software architecture.
The most commonly used types include RESTful APIs, SOAP APIs, and GraphQL APIs
RESTful APIs
Representational State Transfer (REST) APIs are the most widely used in modern web development. They are stateless, meaning each API request from a client contains all the information necessary for the server to fulfill it.
RESTful APIs use standard HTTP methods such as GET, POST, PUT, and DELETE. A well-known example of a RESTful API is the Google Maps API, which allows developers to integrate maps and location services into their applications.
SOAP APIs
Simple Object Access Protocol (SOAP) APIs are more rigid than RESTful APIs and are typically used in enterprise environments where security and transaction integrity are critical.
SOAP uses XML messaging and provides built-in error handling, making it a popular choice for financial and telecommunications industries. Though less common today than REST, SOAP is still valuable in scenarios requiring strict security protocols, as outlined by IBM’s SOAP guidelines.
GraphQL APIs
GraphQL is a newer type of API that allows clients to specify exactly what data they need from an API.
Instead of receiving a large chunk of data, clients can query only the necessary information. This reduces over-fetching and makes the API more efficient.
Facebook originally developed GraphQL to handle the vast amounts of data processed on its platform. Learn more about GraphQL.
Benefits of Using APIs
From improving efficiency to enabling flexibility and scalability, APIs provide a wide range of benefits, making them a crucial part of modern software development.
APIs allow developers to create more robust applications by allowing them more:
Efficiency: APIs significantly speed up the development process by allowing developers to leverage existing services and functionalities.
For instance, rather than building a payment system from scratch, developers can integrate a third-party payment API like Stripe.
This reusability reduces the time and effort required to build complex systems and enables developers to focus on creating unique features for their applications.
Scalability: APIs make scaling applications easier by breaking down systems into smaller, more manageable components.
When an application is modular and API-driven, it becomes simpler to update, expand, or replace individual parts without affecting the entire system.
This decoupling of components allows for more efficient handling of traffic spikes and system growth.
Flexibility: APIs give developers the flexibility to choose the best-in-class tools or services for their specific needs.
For example, a developer can use one API for mapping (e.g., Google Maps API), another for messaging (e.g., Twilio API), and yet another for payments (e.g., PayPal API).
This modularity allows for greater customization and optimization of the application’s features.
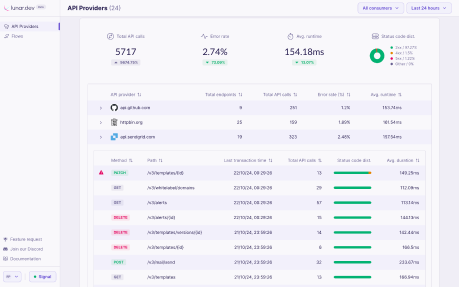
Using APIs in the wild: real world examples
APIs are at the heart of many applications that people use every day.
From social media platforms to e-commerce sites,
APIs power the interactions between users and services. Here are some popular examples of real-world APIs and how they are used:
Google Maps API: The Google Maps API allows developers to integrate mapping and location services into their applications.
This API provides features like real-time traffic updates, directions, and the ability to embed interactive maps on websites or mobile apps. Companies like Uber and Airbnb use the Google Maps API to enhance user experiences by offering location-based services.

Twitter API: The Twitter API gives developers access to Twitter’s vast repository of data, including tweets, user information, and trends.
By using this API, developers can build applications that analyze social media trends, display live tweet feeds, or even schedule automated tweets. Twitter’s API is widely used for both analytical and engagement purposes.
Stripe API: Stripe provides a secure API for processing online payments. This API allows developers to accept credit cards, handle subscriptions, and manage transactions without having to deal with the complexities of building a payment gateway from scratch. It is widely used by e-commerce platforms and mobile applications for secure payment processing.
Facebook API: The Facebook API allows developers to integrate social login, sharing, and other Facebook features into their websites and apps. This API is often used for creating social media-sharing buttons, enabling users to log into an app with their Facebook credentials, and accessing user data (with permission) for targeted advertising and engagement.
How to Implement an API
Implementing an API into your application is a straightforward process, but it requires careful attention to detail, particularly with security and data management.
Here's a very high-level overview of the steps a developer will take to integrate an API into their system:
Step 1: Select an API: First, identify the API that best meets your application’s needs. For example, if you need to display maps, choose a service like Google Maps API. If you need payment processing, consider Stripe or PayPal APIs. Research the API documentation to understand its features and how it works.
Step 2: Obtain API Credentials: Most APIs require you to sign up and create an account to get an API key or other credentials. This key is used to authenticate your application when making requests to the API. Ensure that you store these credentials securely.
Step 3: Make an API Request: Once you have the API key, you can start making requests to the API. Use an HTTP method (GET, POST, PUT, or DELETE) to interact with the API. For example, a GET request retrieves data, while a POST request sends data to the server. The API documentation will provide the correct endpoints and parameters.
Step 4: Handle the Response: APIs typically return data in a format like JSON or XML. Your application will need to parse this response and display or process the data as needed. For example, a weather API might return a JSON object containing temperature and humidity, which your app will then display to the user.
Step 5: Example Code: Here’s an example of making a simple GET request to an API in JavaScript using the fetch function:
This example sends a GET request to an API endpoint and handles the response by logging the data to the console.
The Future of APIs
APIs are set to play an even more significant role in the future of software development, especially in areas like cloud computing, microservices, and machine learning.
Cloud Integration: APIs will continue to be essential in cloud environments, facilitating seamless integration across platforms. As businesses increasingly migrate to the cloud, APIs will serve as the glue that holds different cloud-based services together.
Microservices Architecture: APIs are vital for microservices, where applications are split into smaller, loosely coupled services. Each service communicates with others through APIs, allowing for independent development, scaling, and deployment.
Machine Learning APIs: Machine learning APIs, such as those offered by Google Cloud AI, are enabling developers to integrate advanced machine learning capabilities into their applications without needing deep expertise in AI. This
API Consumption Management: As more services and applications rely heavily on APIs to function, the management of API consumption has become a critical factor for businesses and developers alike.
This concept refers to the strategies and tools used to monitor, control, and optimize how APIs are being used by internal and external consumers.
APIs often have usage limits or quotas, especially when provided by third-party services.
These limits prevent overuse of resources, ensure fair usage, and protect the provider’s infrastructure from excessive demand.
Without proper management, high API usage can lead to unexpected costs, degraded performance, or denial of service.
So what is an API- a conclusion
APIs have become the backbone of modern software development, enabling seamless communication between applications, services, and platforms.
Whether used to integrate third-party services like payment processing, mapping, or social media, APIs empower developers to leverage external functionalities without reinventing the wheel.
Looking ahead, the role of APIs will continue to grow, especially as cloud computing, microservices, and AI APIs gain prominence.
As more businesses adopt API-driven architectures, managing API consumption, security, and performance will become increasingly important.
APIs are not just a tool for developers; they are a strategic enabler for innovation, collaboration, and the growth of the digital economy.
Ready to Start your journey?
Manage a single service and unlock API management at scale